Professional training
Backend Development
in Java: From Zero to PRO
in Java: From Zero to PRO
You will master all key aspects of Java, including the basics of the language and prepare yourself to
tackle complex backend development tasks.
Start now
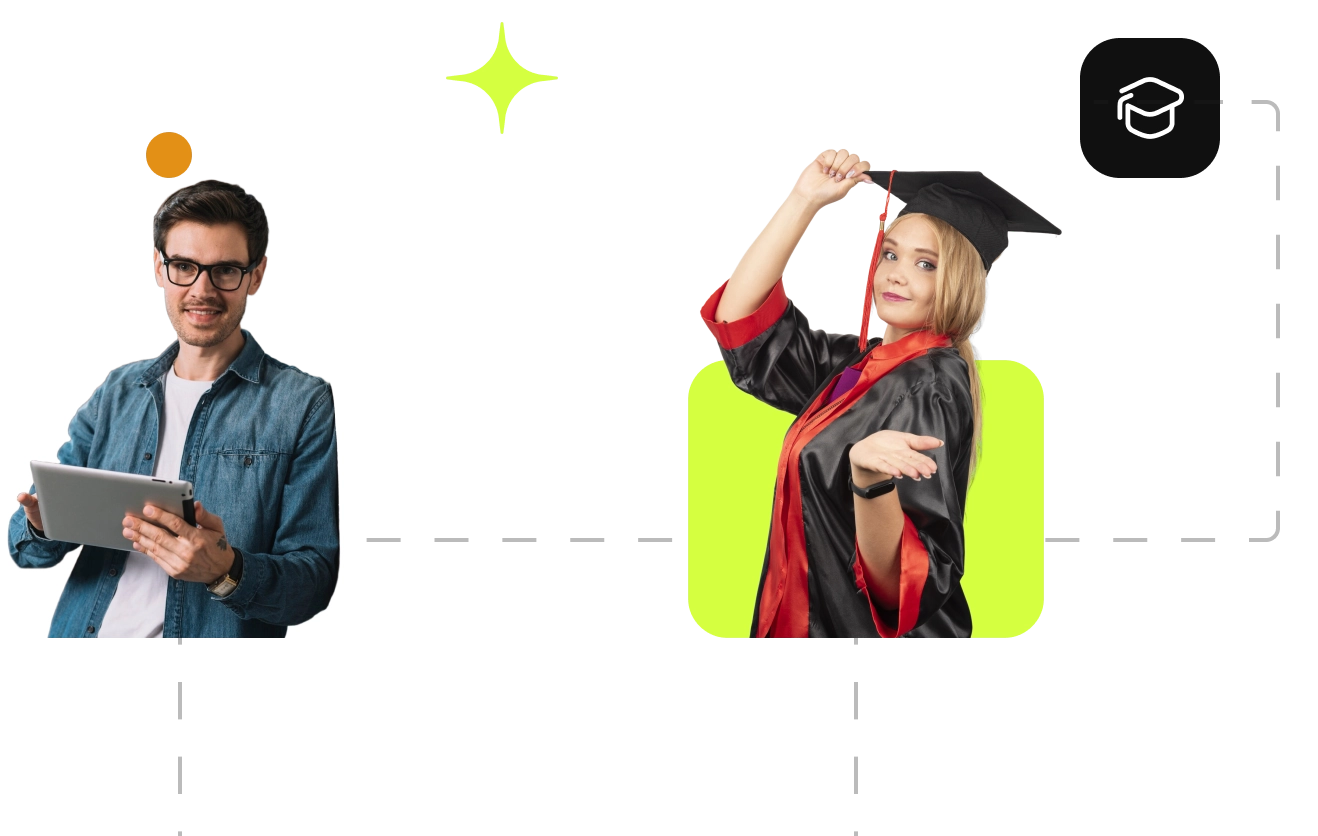